Programming in Kotlin
Mar 20 2018 · Video Course (4 hrs, 2 mins) · Beginner
Take a deep dive into the Kotlin language, learning about core Kotlin concepts like types, Nullables, functions, classes, and more.
3/5Version
- Kotlin 1.2, IntelliJ IDEA 2017.3
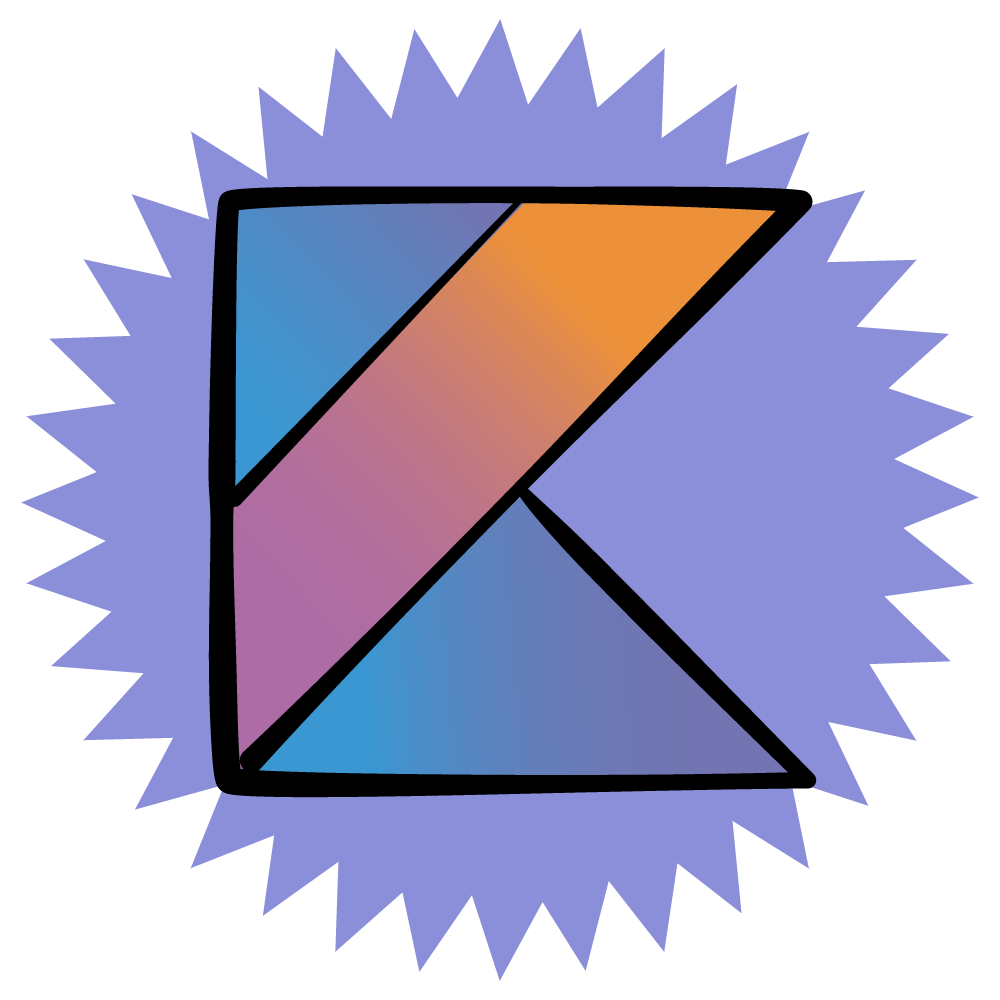
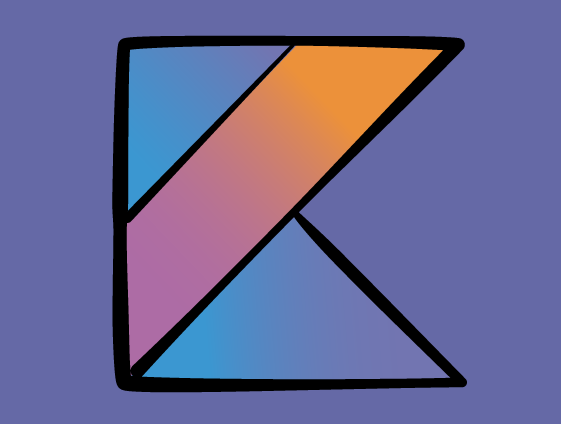
Core Concepts
Let's review what you'll be learning in this section, and why it's important.
1Using Intellij
3:29Install IntelliJ IDEA, then take a tour of the IDE and create the starter project you'll throughout this course.
2Kotlin Basics
6:24Review some of the core concepts of Kotlin that were covered in Your First Kotlin Android App course.
3Comments
1:35Learn the various ways to add comments to your Kotlin code - a useful way to document your work or add notes for future reference.
4Learn about two Kotlin classes, Pair and Triple, and get tips about when you should use each of them.
5In this video, practice using the Pair and Triple classes on your own through a hands-on challenge.
6Learn what the concept of scope means in Kotlin, and how it applies to if statements.
9Conclusion
1:10Let's review where you are with your Kotlin core concepts, and discuss what's next.
10Flow Control
While Loops
3:05Learn how to make Kotlin repeat your code multiple times with while loops, repeat while loops, and break statements.
12Practice using while loops on your own, through a hands-on challenge.
13For Loops
5:23Learn how to use for loops in Kotlin, along with ranges, continue, and labeled statements.
14Learn how to use when expressions in Kotlin, including some of its more powerful features.
16Practice using when expressions on your own, through a hands-on challenge.
17Functions and Nullables
Write your own functions in Kotlin, and see for yourself how Kotlin makes them easy to use.
20Practice writing functions on your own, through a hands-on challenge.
21More Functions
8:12More Functions: Learn some more advanced features of functions, such as overloading, and functions as variables.
22Learn about one of the most important aspects of Kotlin development - nullables - through a fun analogy.
23Practice using Nullables on your own, through a hands-on challenge.
24More Nullables
6:17Learn how to unwrap Nullables, force unwrap Nullables, and use the let statement.
25Practice working with Nullables on your own, through a hands-on challenge.
26Conclusion
0:55Let's review where you are with your Kotlin core concepts, and discuss what's next.
27Collections
Find out which collection you should use in a given situation.
35Lambdas
Learn the difference between lambdas and higher-order functions.
39Classes
Introduction
0:57Let's review what you'll be learning in the Classes section, and why it's important.
46Challenge: Creation
8:25Practice creating classes and understanding when to use them through a hands-on challenge.
49Inheritance
15:45Learn about the concepts of inheritance, polymorphism, hierarchy checks, overrides, and super.
50Conclusion
0:49Let's review where you're at with your Kotlin core concepts, and discuss what's next.
54More on Classes
Practice working with creating singleton classes and understanding when to use them, through a hands-on challenge.
58Conclusion
0:33Let's review where you're at with your Kotlin core concepts, and discuss what's next.
65Properties and Methods
Introduction
0:30Let's review what you'll be learning in the Properties and Methods section, and why it's important.
66Learn about getters and setters, and why you don’t need to create them in most cases.
67Challenge time! Practice using properties through a hands-on challenge.
73Conclusion
1:55Congratulations, you made it through the whole course! Let's review what we've learned.
74
Comments